Public Shared Function Format( _
ByVal Expression As Object, _
Optional ByVal Style As String = "" _
) As String
As mentioned above, this function can be used in various
scenarios. The simplest way to use this function is to pass it a number, a
string, or a date/time). The function would then produce that number.
Besides the Format() function, the Visual Basic
language provides some additional functions we will review below.
So far, to display a number, we simply passed it to the MsgBox()
function or to another procedure. In some cases, you may want the number to
display in a particular format. To control how the number should display, you
can pass the second argument of the Format() function. This argument
would be passed as a string.
To produce the number in a general format, you can pass the
second argument as "g", "G", "f",
or "F" .
To display the number with a decimal separator, pass the
second argument as "n", "N", or "Standard".
Here is an example:
Public Module Exercise
Public Function Main() As Integer
Dim Number As Double
Number = 20502.48
MsgBox("Number: " & Format(Number, "STANDARD"))
Return 0
End Function
End Module
This would produce:
![]()
An alternative to get this format is to call a function
named FormatNumber. Its syntax is:
Function FormatNumber( ByVal Expression As Object, Optional ByVal NumDigitsAfterDecimal As Integer = -1, Optional ByVal IncludeLeadingDigit As TriState = TriState.UseDefault, Optional ByVal UseParensForNegativeNumbers As TriState = TriState.UseDefault, Optional ByVal GroupDigits As TriState = TriState.UseDefault ) As String
Only the first argument is required and it represents the
value to display. If you pass only this argument, you get the same format as the
Format() function called with the Standard option. Here is an
example:
Public Module Exercise
Public Function Main() As Integer
Dim Number As Double
Number = 20502.48
MsgBox("Number: " & Format(Number, "STANDARD"))
MsgBox("Number: " & FormatNumber(Number))
Return 0
End Function
End Module
This would produce the same result as above.
If you call the Format() function with the Standard
option, it would consider only the number of digits on the right side of the
decimal separator. If you want to display more digits than the number actually
has, call the FormatNumber() function and pass a second argument with the
desired number. Here is an example:
Public Module Exercise
Public Function Main() As Integer
Dim Number As Double
Number = 20502.48
MsgBox("Number: " & Format(Number, "STANDARD") & vbCrLf & _
"Number: " & FormatNumber(Number, 4))
Return 0
End Function
End Module
This would display:
![]() |
||||||||||||
|
In the same way, if you want the number to display with less
numbers on the right side of the decimal separator, specify that number.
As a third alternative, you can call the Format()
function. In reality, the second argument is used to format the number with many
more options. To represent the integral part of a number, you use the # sign. To
specify the number of digits to display on the right side of the decimal
separator, type a period on the right side of # followed by the number of 0s
representing each decimal place. Here is an example:
Public Module Exercise
Public Function Main() As Integer
Dim Number As Double
Number = 20502.48
MsgBox("Number: " & Format(Number, "#.00000"))
Return 0
End Function
End Module
The five 0s on the right side of the period indicate that
you want to display 5 digits on the right side of the period. This would
produce:
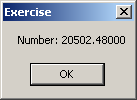
You can enter as many # signs as you want; it wouldn't
change anything. Here is an example:
Public Module Exercise
Public Function Main() As Integer
Dim Number As Double
Number = 20502.48
MsgBox("Number: " & Format(Number, "##########.00000"))
Return 0
End Function
End Module
This would produce the same result as above. To specify that
you want to display the decimal separator, include its character between the #
signs. Here is an example:
Public Module Exercise
Public Function Main() As Integer
Dim Number As Double
Number = 20502.48
MsgBox("Number: " & Format(Number, "###,#######.00000"))
Return 0
End Function
End Module
This would produce:

You can include any other character or symbol you want in
the string to be part of the result, but you should include such a character
only at the beginning or the end of the string, otherwise the interpreter might
give you an unexpected result.
Tidak ada komentar:
Posting Komentar